In this article we will see an introduction to the main features of Dash, a basic tutorial with a practical example, and an overview of some of its more advanced features.
What is Dash and what is it for
Dash is anopen-source library for Pythonthat allows you tocreate interactive data-drivenWeb applications.
One of the main features of Dash is itsReact.js-based architecture. This enables the creation of responsive web applications with a smooth user experience.
Dash also includes the Plotly library for data visualization, which means that interactive and animated charts can be created easily and efficiently.
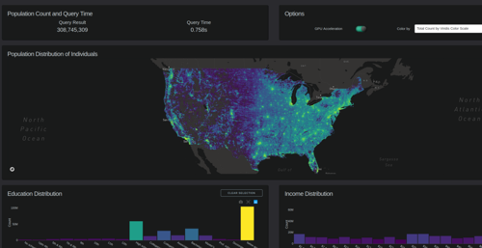
The library is available for Python 3 and is easy to install via pip. Dash provides numerous predefined HTML and CSS components, which enable the creation of sophisticated user interfaces. In addition, Dash offers a wide range of customization options and integration with other frameworks such as Flask.
Dash is a very useful library for Data Analysts and Data Scientistswho wish to create web applications to share their results with others or to create interactive dashboards for data visualization.
Python Dash Basic Tutorial
Let us now look at a Dash tutorial with a practical example.
In this tutorial, we will create a simple web application that allows the user to select a country and view the number of gold, silver, and bronze medals won by that country at the 2021 Summer Olympics.
Step 1: Install and import the necessary libraries
To get started, you need to install the Dash library.
We open the command prompt or terminal and type:
pip install dash
After installing Dash, we import the necessary libraries into our script:
import dash
import dash_core_components as dcc
import dash_html_components as html
import pandas as pd
dash is the core library of Dash, dash_core_components contains core components such as input, output, dropdown, etc., while dash_html_components contains HTML components such as div, H1, p, etc. pandas will be used to load data from csv file.
Step 2: Loading data
We create a CSV file with the 2021 Olympic medal data.
The data will be organized in this way:
Country Gold Silver Bronze
Italy 10 10 20
United States 20 30 10
Japan 30 20 10
… … … …
We save the file as “medals.csv” in the same folder where the script is located.
After creating the file, we can load it into our script using Pandas:
medals_df= pd.read_csv(‘medals.csv’)
Step 3: Creating the application
To create our application, we need to create an instance of the dash.Dash() object and define the layout of the application using HTML and Dash core components.
In this example, we will create an application with a dropdown that allows the user to select a country and view the number of gold, silver, and bronze medals won by that country.
app= dash.Dash(__name__)
app.layout= html.Div([
dcc.Dropdown(
id=’country-dropdown’,
options=[{‘label’:country, ‘value’:country} for country in medals_df[‘Country’]],
value=’Italy’
),
html.H1(id=’medals-header’),
html.Div([
html.Div([
html.H2(‘Gold’),
html.Div(id=’gold-medals’)
], className=’medal’),
html.Div([
html.H2(‘Silver’),
html.Div(id=’silver-medals’)
], className=’medal’),
html.Div([
html.H2(‘Bronze’),
html.Div(id=’bronze-medals’)
], className=’medal’)
], className=’medals-container’)
])
In the first line of the layout, we have a dropdown with the id “country-dropdown, “ which allows the user to select the country. The dropdown options are generated based on the countries in our dataframe.
The next line is an HTML header with id “medals-header, “ which will be used to display the name of the country selected by the user.
In the next block, we create three divs with class “medal” inside a div with class “medals-container.” Each div contains an HTML header with the name of the metal and a div with an id that we will use to display the number of medals.
Step 4: Defining callback functions
To update the medal numbers when the user selects a new country, we need to define some callback functions.
Specifically, we need to define a function that updates the HTML header with the name of the selected country and three functions that update the divs with medal numbers.
@app.callback(
dash.dependencies.Output(‘medals-header’,’children’),
[dash.dependencies.Input(‘country-dropdown’, ‘value’)]])
defupdate_header(selected_country):
return f’Medals won by{selected_country}’
@app.callback(
dash.dependencies.Output(‘gold-medals’,’children’),
[dash.dependencies.Input(‘country-dropdown’, ‘value’)]])
defupdate_gold_medals(selected_country):
gold = medals_df.loc[medals_df[‘Paese’] ==selected_country, ‘Gold’].iloc[0]
return f'{gold}’
@app.callback(
dash.dependencies.Output(‘silver-medals’,’children’),
[dash.dependencies.Input(‘country-dropdown’, ‘value’)]])
defupdate_silver_medals(selected_country):
silver = medals_df.loc[medals_df[‘Paese’]== selected_country, ‘Silver’].iloc[0]
return f'{silver}’
@app.callback(
dash.dependencies.Output(‘bronze-medals’,’children’),
[dash.dependencies.Input(‘country-dropdown’, ‘value’)]])
defupdate_bronze_medals(selected_country)
bronze = medals_df.loc[medals_df[‘Paese’]== selected_country, ‘Bronze’].iloc[0]
return f'{bronze}’
Each callback function is decorated with the @app.callback annotation, which specifies the output that the function should update and the inputs that should trigger the function
Step 5: Running the application
To run the application, we can add the following code to the end of our script:
if__name__ == ‘__main__’:
app.run_server(debug=True)
This starts the development server and opens the application in the default browser.
Advanced features of Dash
Dash is an extremely flexible and customizable library that can support a wide range of advanced features for creating interactive Web applications.
Let’s look below at some of the main advanced features it offers.
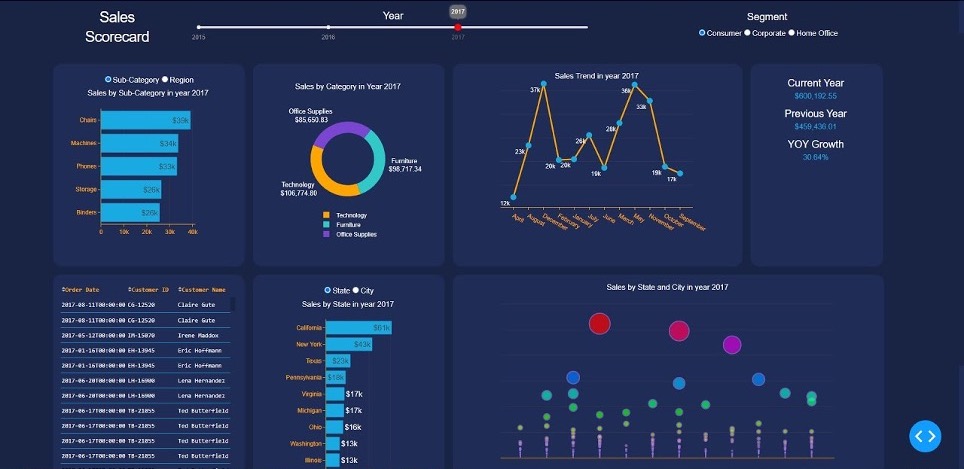
Integration with Plotly
Dash is built onPlotly, an interactive data visualization library that supports a wide range of chart types. Dash makes it easy to incorporate Plotly charts into web applications.
Advanced interactivity features
Dash enablescomplex interactionsbetween application components, such as updating a graph based on user selections or filtering data in real time.
Support for state management
Dash allows you tomanage application status, such as keeping track of user selections or data changes. This enables the creation of web applications that provide a more personalized and consistent user experience.
Appearance customization
Dash allows you to customize the appearance of your application usingcustom CSS style sheets. This allows you to create web applications with a customized user experience that is consistent with your company’s brand.
Support for animations
Dash supports animations, allowing you to create web applications with eye-catching visual effects that enhance the user experience.
File Management
Dash allows users toupload, save and download filesusing components such as dcc.Upload and dcc.Download. This enables the creation of web applications that allow users to interact with files in a more intuitive way.
Support for data caching
Dash allowscaching of data to improve application performance. This is especially useful when working with large amounts of data or when updating data is time-consuming.
Support for web application sharing
Dash makes iteasy to share web applicationswith others using hosting services such as Heroku or AWS.
Conclusion
These are just some of the features offered by Dash. Because of its flexibility and customization possibilities, Dash can be used to create a wide range of interactive Web applications, from business intelligence dashboards to scientific applications.